in handphone apps:
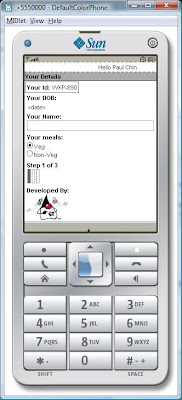
import javax.microedition.midlet.MIDlet;
import javax.microedition.lcdui.*;
public class DateTimeApp2 extends MIDlet {
private Form form;
private Gauge gauge;
//private Spacer spacer;
private ImageItem imageItem;
private TextField txtField;
private DateField dateField;
private StringItem stringItem;
private ChoiceGroup choiceGroup;
public DateTimeApp2() {
form = new Form("Your Details");
form.setTicker(new Ticker("Hello Paul Chin"));
// a StringItem is not editable
stringItem = new StringItem("Your Id: ", "WXP-890");
form.append(stringItem);
// you can accept Date, Time or DateTime formats
dateField = new DateField("Your DOB: ", DateField.DATE);
form.append(dateField);
// similar to using a TextBox
txtField = new TextField(
"Your Name: ", "", 50, TextField.ANY);
form.append(txtField);
// similar to using a List
choiceGroup = new ChoiceGroup(
"Your meals: ",
Choice.EXCLUSIVE,
new String[] {"Veg", "Non-Veg"},
null);
form.append(choiceGroup);
// put some space between the items to segregate
//spacer = new Spacer(20, 20);
//form.append(spacer);
// a gauge is used to show progress
gauge = new Gauge("Step 1 of 3", false, 3, 1);
form.append(gauge);
// an image may not be found,
// therefore the Exception must be handled
// or ignored
try {
imageItem = new ImageItem(
"Developed By: ",
Image.createImage("/images/Duke.png"),
ImageItem.LAYOUT_DEFAULT,
"DuKe");
form.append(imageItem);
} catch(Exception e) {}
}
public void startApp() {
Display display = Display.getDisplay(this);
display.setCurrent(form);
}
public void pauseApp() {
}
public void destroyApp(boolean unconditional) {
}
}
Note that the Spacer object is unavailable for MIDP1.0 hence
it needs to be commented out. It is only available for MIDP2.0 and
above.
Also be sure to put the Duke.png pic in the images folder:
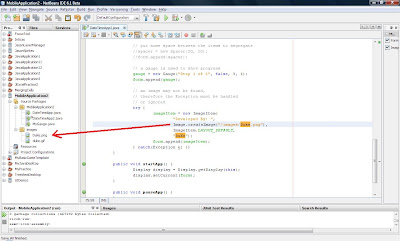
Note that for T610, you need to use .png graphics. T610 cannot
display .gif
If for any reason, your image fails to display, then right click
on Resource and add the images folder path.