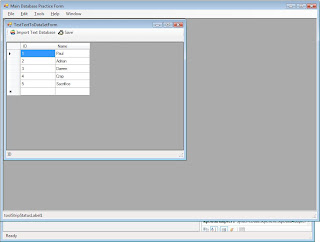
The MDI Child form entitled TestTextToDataSetForm shows a Tooltip button called Import Text Database. When you click on this, the program will read the file called:
C:\Users\Paul\Documents\test2.txt
and display it in the GridView Object.
There is also another button called Save which can save any changes made back to the TXT file.
Insert the child MDI form with the following objects in Document Explorer:
The red arrows above indicate the objects. Insert in this order:
- Dock statusStrip1 at bottom of TestTextToDataSetForm (“the Form”).
- Dock splitContainer1 in Fill mode docking.
- Set the splitContainer1 orientation to Horizontal.
- Reduce splitContainer1.Panel1 to minimum by dragging the separator up.
- Dock toolStrip1 in splitContainer1.Panel1.
- Insert Import Text Database Button (with Apple icon).
- Name it ImportTextDatabaseButton.
- Insert Save Button (Penguin Icon)
- Name it SaveButton.
Then insert event handler for the buttons:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using TestTextToDataSet;
using System.IO;
namespace DataBasePractice
{
public partial class TestTextToDataSetForm : Form
{
string path = @"C:\Users\Paul\Documents\test2.txt";
StringBuilder strDataItem = new StringBuilder();
DataSet ds;
public TestTextToDataSetForm()
{
InitializeComponent();
}
private void ImportTextDatabase_Click(object sender, EventArgs e)
{
ds = TextToDataSet.Convert(path, "MyNewTable", "\t");
dataGridView1.DataSource = ds.Tables[0];
dataGridView1.Update();
dataGridView1.Show();
toolStripStatusLabel1.Text = ds.Tables[0].Columns[0].ToString();
insertColumnNames2string();
}
private void insertColumnNames2string()
{
strDataItem.Remove(0, strDataItem.Length);
int numOfCols = ds.Tables[0].Columns.Count, count = 1;
foreach (DataColumn dataCol in ds.Tables[0].Columns)
{
strDataItem.Append(dataCol.ToString());
if (count < style="color: rgb(163, 21, 21);">"\t");
count++;
}
strDataItem.Append(Environment.NewLine);
}
private void Save_Click(object sender, EventArgs e)
{
int numOfCols = ds.Tables[0].Columns.Count, count = 1;
insertColumnNames2string();
StreamWriter sw;
foreach (DataRow theRow in ((DataTable)dataGridView1.DataSource).Rows)
{
foreach (DataColumn theColumn in
(DataTable)dataGridView1.DataSource).Columns)
{
strDataItem.Append(theRow[theColumn].ToString());
if (count < style="color: rgb(163, 21, 21);">"\t");
count++;
}
strDataItem.Append(Environment.NewLine);
count = 1;
}
sw = new StreamWriter(path);
sw.WriteLine(strDataItem);
sw.Close();
}
}
}
Thanks to Dave, the reference using TestTextToDataSet refers to this class below:
(http://www.codeproject.com/cs/database/DataSetFrmDelimTxt.asp?
df=100&forumid=38170&select=2270400&msg=2270400)
using System;
using System.Collections.Generic;
using System.Text;
using System.Data;
using System.IO;
namespace TestTextToDataSet
{
public class TextToDataSet
{
public TextToDataSet()
{ }
public static DataSet Convert(string File,
string TableName, string delimiter)
{
//The DataSet to Return
DataSet result = new DataSet();
//Open the file in a stream reader.
StreamReader s = new StreamReader(File);
//Split the first line into the columns
string[] columns = s.ReadLine().Split(delimiter.ToCharArray());
//Add the new DataTable to the RecordSet
result.Tables.Add(TableName);
//Cycle the colums, adding those that don't exist yet
//and sequencing the one that do.
foreach (string col in columns)
{
bool added = false;
string next = "";
int i = 0;
while (!added)
{
//Build the column name and remove any unwanted characters.
string columnname = col + next;
columnname = columnname.Replace("#", "");
columnname = columnname.Replace("'", "");
columnname = columnname.Replace("&", "");
//See if the column already exists
if (!result.Tables[TableName].Columns.Contains(columnname))
{
//if it doesn't then we add it here and mark it as added
result.Tables[TableName].Columns.Add(columnname);
added = true;
}
else
{
//if it did exist then we increment the sequencer and try again.
i++;
next = "_" + i.ToString();
}
}
}
//Read the rest of the data in the file.
string AllData = s.ReadToEnd();
//Split off each row at the Carriage Return/Line Feed
//Default line ending in most windows exports.
//You may have to edit this to match your particular file.
//This will work for Excel, Access, etc. default exports.
string[] rows = AllData.Split("\r\n".ToCharArray());
//Now add each row to the DataSet
foreach (string r in rows)
{
//Split the row at the delimiter.
string[] items = r.Split(delimiter.ToCharArray());
//Paul: Solves blank row import problem
if (r != "")
{
//Add the item
result.Tables[TableName].Rows.Add(items);
}
}
//Return the imported data.
return result;
}
}
}